一直想找notadd的后台时如何创建路由的,结果一直没有找到
今天总算是让我找到了
一下记录一下步骤
获取所有路由列表
php notadd route:list > D:route.txt
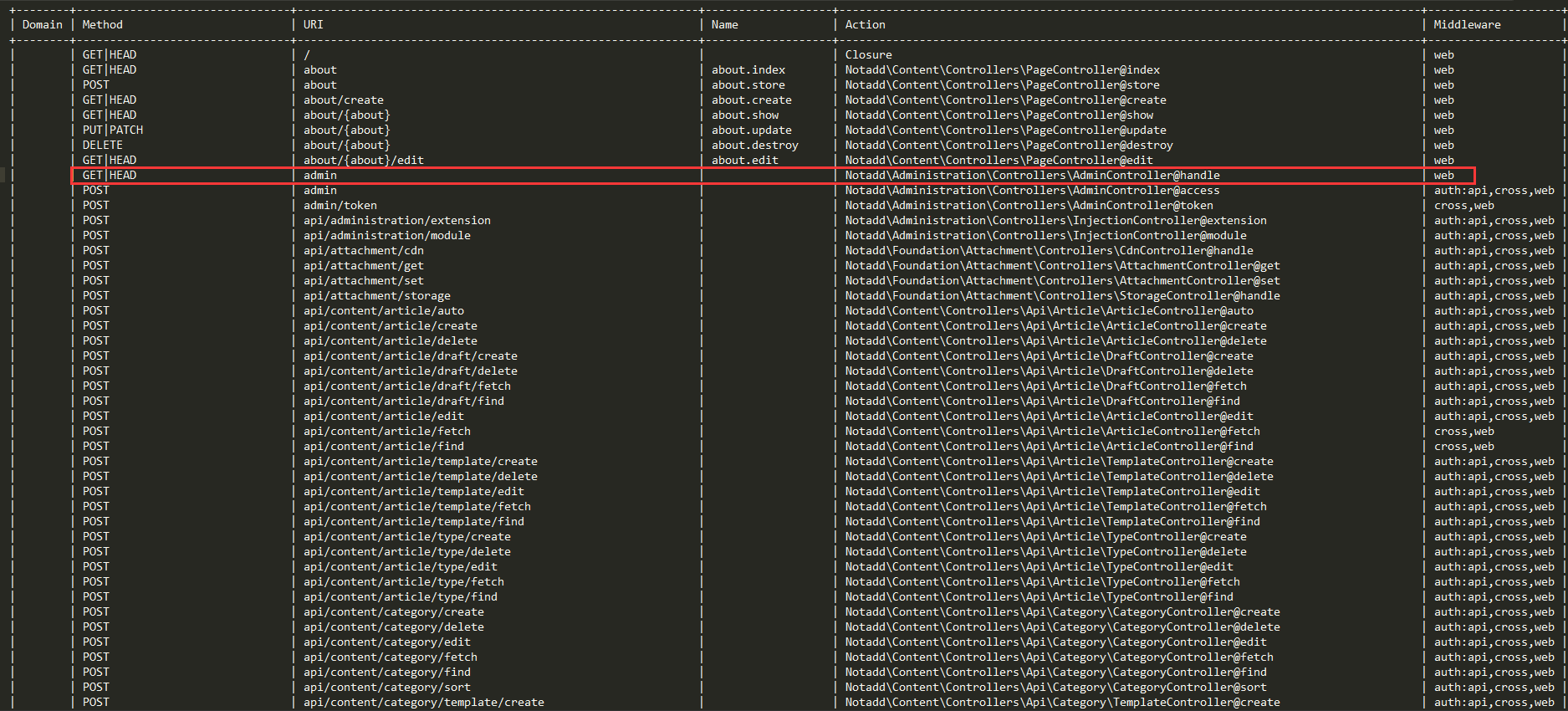
发现是在Notadd\Administration\Controllers\AdminController这个文件里定义的
我打开notadd\modules\administration\src\ModuleServiceProvider.php
看到ModuleServiceProvider的boot function是这样的
/**
* Boot service provider.
*
* @throws \Illuminate\Contracts\Container\BindingResolutionException
*/
public function boot()
{
$administrator = new Administrator($this->app['events'], $this->app['router']);
$administrator->registerPath('admin');
$administrator->registerHandler(AdminController::class . '@handle');
$this->administration->setAdministrator($administrator);
$this->app->make(Dispatcher::class)->subscribe(CsrfTokenRegister::class);
$this->app->make(Dispatcher::class)->subscribe(PermissionGroupRegister::class);
$this->app->make(Dispatcher::class)->subscribe(PermissionModuleRegister::class);
$this->app->make(Dispatcher::class)->subscribe(PermissionRegister::class);
$this->app->make(Dispatcher::class)->subscribe(PermissionTypeRegister::class);
$this->app->make(Dispatcher::class)->subscribe(RouteRegister::class);
$this->loadTranslationsFrom(realpath(__DIR__ . '/../resources/translations'), 'administration');
$this->loadViewsFrom(realpath(__DIR__ . '/../resources/views'), 'admin');
$this->publishes([
realpath(__DIR__ . '/../resources/mixes/administration/dist/assets/admin') => public_path('assets/admin'),
realpath(__DIR__ . '/../resources/mixes/neditor') => public_path('assets/neditor'),
], 'public');
}
重点是这几行

我不知道registerPath和registerHandler是做什么用的,那么我向上追溯到了notadd\vendor\notadd\framework\src\Administration\Abstracts\Administrator.php
/**
* Init administrator.
*
* @throws \InvalidArgumentException
*/
final public function init()
{
if (is_null($this->path) || is_null($this->handler)) {
throw new InvalidArgumentException('Handler or Path must be Setted!');
}
$this->router->group(['middleware' => 'web'], function () {
$this->router->get($this->path, $this->handler);
});
}
/**
* Register administration handler.
*
* @param $handler
*/
public function registerHandler($handler)
{
$this->handler = $handler;
}
/**
* Register administration route path.
*
* @param string $path
*/
public function registerPath($path)
{
$this->path = $path;
}
发现了这些代码,好吧 原来这个路由是在这里去绑定的······ 怪不得我开始怎么找也找不到呢
所以是吧/admin 绑定到了notadd\modules\administration\src\Controllers\AdminController.php 的 handle function
/**
* Return index content.
*
* @param \Notadd\Foundation\Extension\ExtensionManager $extension
* @param \Notadd\Foundation\Module\ModuleManager $module
*
* @return \Illuminate\Contracts\View\View
*/
public function handle(ExtensionManager $extension, ModuleManager $module)
{
$this->share('extensions', $extension->getEnabledExtensions());
$this->share('modules', $module->getEnabledModules());
$this->share('translations', json_encode($this->translator->fetch('zh-cn')));
return $this->view('admin::layout');
}
所以在这些操作之前,一定有一个地方初始化了所有的module
我想找到这个地方